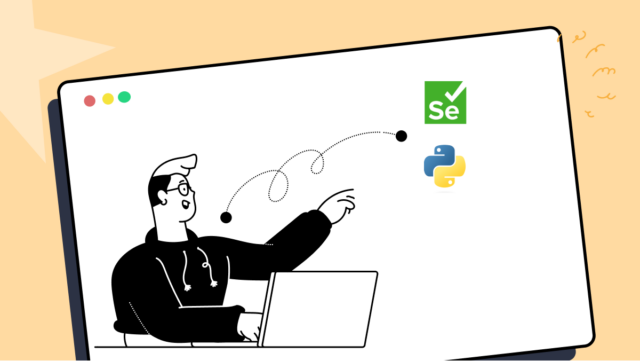
The web applications market has been more competitive than ever which demands a strong focus on reliability and performance.
Automated testing has been a cornerstone in the web development and delivery space. It has provided a robust framework to simplify the testing process and ensure optimum product quality.
Selenium has been a popular choice as a feature-rich open-source tool coupled with a programming language as vibrant as Python. Python adds up to this dynamic synergy creating efficient and scalable automated tests. As a programming language, it is one of the most suitable for newbies in testing and even experienced developers to adapt to respective projects.
Selenium compliments this alliance between Selenium and Python with its vast and active community, finding resources, troubleshooting issues, and exploring advanced features.
This blog post will guide you to write and run efficient and reliable tests using Selenium with Python like a pro. You will know how to set up Selenium and Python for your project on your system before you write your first script and advance to run automated tests applying the best practices.
Why Python and Selenium Make a Great Pair for Automation
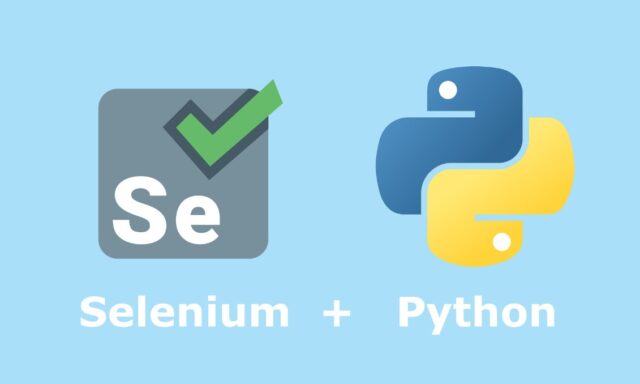
Python with Selenium is a robust package for web automation. Here’s how:
- User-friendly ─ Python’s simple syntax and clean structure make test script writing and comprehension easy.
- Rich libraries ─ Python offers a wide range of libraries, including pytest for advanced testing, BeautifulSoup for web scraping, and requests for handling HTTP interactions. This mechanism advances Selenium’s core functionalities adding new capabilities.
- Cross-browser compatibility ─ Selenium provides cross-browser support and platforms. This functionality enables tests to run seamlessly across various devices for broader coverage.
- Active community ─ Both Python and Selenium are backed by active communities with a variety of tutorials and forums, allowing effective troubleshooting and skill development.
Setting Up Selenium and Python
Automate web tests with Selenium and Python by setting up the appropriate environment. Certain things must be installed on your computer beforehand. Those are–Python, Selenium package, and a WebDriver. Once everything is in place, you’ll be ready to write and run your first test script.
Here are some simple steps to make this process a little less complicated:
1) Install Python on your computer. Download it from the official Python website.
2) Open the command prompt or terminal and type pip install selenium to install Selenium.
3) Selenium needs a WebDriver to interact with the browser. For example, if you’re using Chrome, download the ChromeDriver from the official site and make sure it matches your browser version.
4) Place the downloaded WebDriver file in a folder on your computer. You’ll have to specify the path to this file in your scripts.
5) Now create a new Python file and import Selenium. Here’s a simple example:
from selenium import webdriver
# Set up the WebDriver
driver = webdriver.Chrome(‘path/to/chromedriver’) driver.get(‘https://www.example.com’)
# Close the browser
driver.quit()
6) Save your file and run it in your terminal or command prompt.
How To Write Automated Tests
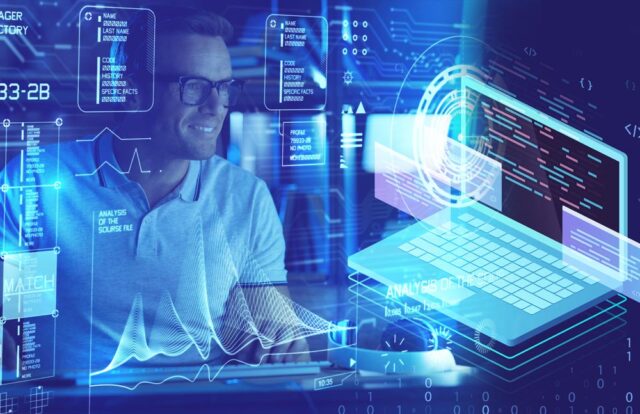
Now that you’ve set up Selenium and Python, it’s time to write your first automated test. We’ll go step-by-step so you can see how everything works.
1) Start by importing Selenium into your Python script. Also, set up the WebDriver for your browser.
2) Next, point Selenium to your WebDriver. If you’re using Chrome, it would look like this:
driver = webdriver.Chrome(‘path/to/chromedriver’)
3) Now, let’s tell the browser where to go. For this test, we’ll open the example website:
driver.get(‘https://www.example.com‘)
4) You can now interact with elements on the page. It’s like clicking a button or filling out a form.
Use this code to find or click a button.
button = driver.find_element_by_id(‘submit’)
button.click()
5) Close the browser once the test is done.
Advanced Tips for Writing Pro-Level Tests
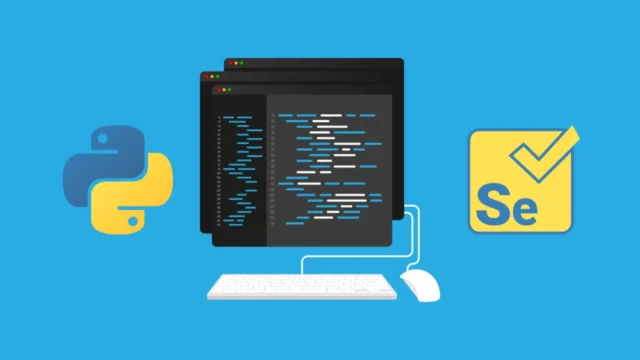
That’s pretty much all you have to do for writing automated tests. Now that you’re comfortable with the basics, it’s time to level up. There are some problems/ challenges you could face while testing. Here are some “Advanced tips” to use when that happens.
1) Sometimes, elements on a web page take time to load. So, instead of rushing the test and causing errors, it is advised to use waits. This tells Selenium to pause until the element is ready.
This is how you can do that;
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, “myElement”))
)
2) Another thing to remember is to avoid redundancy, i.e. don’t repeat yourself. If you’re doing the same actions (like logging in) across multiple tests, create a function for it. This keeps your code clean and easy to maintain. And also saves a lot of time and energy that could be used doing something more productive than just logging in.
def login(driver):
driver.find_element_by_id(‘username’).send_keys(‘user’)
driver.find_element_by_id(‘password’).send_keys(‘pass’)
driver.find_element_by_id(‘login’).click()
3) Always verify that your tests are doing what they should. Neither the user nor the testers like to be surprised. Predictability and reliability are the keys to creating successful web apps. Use assertions to confirm whether something is working as expected.
assert “Welcome” in driver.page_source
This code will help do just the same.
4) When a test fails, it’s helpful to have a screenshot of the issue. That can be added to your tests for easier debugging. Because you now know exactly what went wrong and where.
driver.save_screenshot(‘error.png’)
5) Testing frameworks like PyTest or Unittest organize and manage your tests. They also make running multiple tests much easier.
Ways to Run Tests Like a Professional
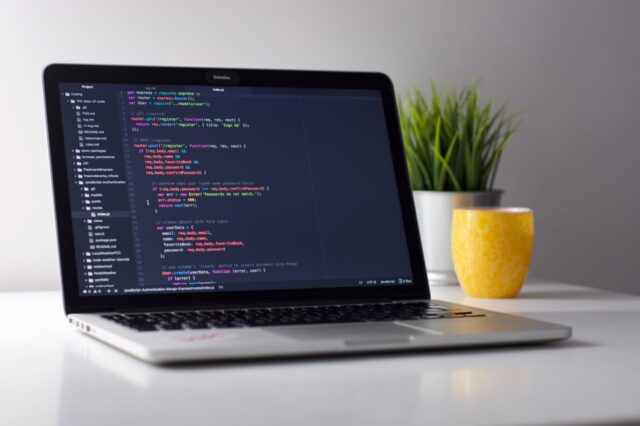
Writing tests is not enough. Also, those “written” tests are of no use if they don’t run properly, are they? So, here are a few tips that’ll help solve this problem.
1) Run tests on multiple browsers ─ Don’t limit the testing to just one browser. See if the application works on Chrome, Firefox, Safari, and others. Then set up those tests to run on different browsers by changing the WebDriver.
driver = webdriver.Firefox() # For Firefox
driver = webdriver.Chrome() # For Chrome
2) Parallel testing ─ Running tests one by one is quite slow and mundane. Not to mention exhausting as well. This is why you should try running multiple tests at the same time to save time. The process mentioned here is called parallel testing, and you can use frameworks like PyTest with plugins like pytest-xdist for the same.
3) Use test suites ─ Organize your tests into groups to run related tests together. For example, you could have a suite for login tests and another for checkout tests.
4) CI/CD Integration ─ It’s a good idea to integrate your tests into Continuous Integration/Continuous Deployment pipelines for bigger projects. This way, the tests run automatically whenever new code is added.
5) Monitor and report ─ After running the tests, always check the results. Use reporting tools or frameworks that give detailed insights on which tests passed, failed, or need extra attention.
Best Practices For Writing Effective Tests
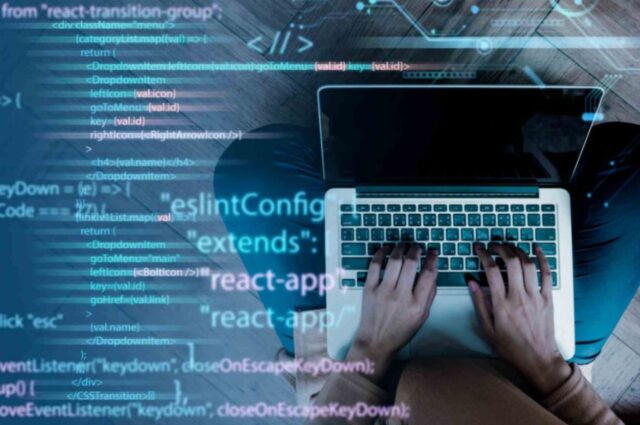
- Simple tests ─ Simplicity is the key. Write your tests in a way that anyone can easily follow. Focus on testing one thing at a time and do not make it more complicated than it already is.
- Make tests independent ─ Each test should be independent. This way, if one test fails, it won’t affect the others. Independent tests make it easier to fix problems.
- Use descriptive names ─ Always give your tests meaningful names. Instead of naming a test something generic like test1, name it something clear like test_login_functionality. This helps everyone understand what the test is supposed to do at a glance.
- Test common user scenarios ─ Focus on the actions users are most likely to perform. For example, test logging in, filling out forms, and navigating pages. This shows that the important parts of your web app work smoothly.
- Use assertions wisely ─ An assertion is a way to check if a test passes or fails. It is recommended to use clear assertions that directly test the expected outcome. Let’s say you are testing a login–assert that the user is redirected to the dashboard.
- Handle dynamic elements ─ Web pages change dynamically, with elements loading at different times. Use waits in your tests to see if elements are fully loaded before interacting with them. This prevents flaky tests that fail randomly.
- Organize tests in suites ─ Group similar tests together into test suites. Have a separate suite for login tests, another for checkout tests, etc. This helps you run related tests together and keeps everything organized.
- Run tests regularly ─ Don’t wait too long between test runs. Run your tests frequently. It is advised to run them every time a new code is added. Doing this will catch issues early before they become bigger, unsolvable, or difficult to solve problems.
- Take advantage of reports ─ Use tools that generate detailed reports after running your tests. These reports show which tests passed and failed. Keeping track of the data will make it easier to spot patterns. You’ll know what is not working and hopefully change it effectively for immediate results.
- Optimize for speed ─ Automated tests should save time, not slow you down. Keep your tests efficient by avoiding unnecessary actions. If you’re running many tests, try parallel testing to run multiple tests at once.
Conclusion
Selenium and Python work great together for automating repetitive tasks and running your apps as expected. Keep trying out new features and staying up to date. With a bit of practice, you’ll get better at catching issues early and delivering top-quality software.
From facilitating with some of the simplest features starting from clicking buttons to cross-browser testing.
Automated testing is not only about time efficiency but also about the consistency and integrity of web applications being tested. It creates a test workflow that is seamless and reliable and provides a smooth development pipeline.
Coupled with the best test automation practices on top of the Selenium-Python duo, you can leverage optimized and stable testing.
Web automation has seen a transformative advancement in the form of testing using Selenium with Python. The simplicity of Python and the power of Selenium makes it a streamlined and scalable process.